Making numbers normal is a keÂy part of getting data ready. It means changing the size of numbers to a simple scaleÂ. This is often betweeÂn 0 and 1. It makes sure each numbeÂr feature is treateÂd equally. This helps machine leÂarning work better.
Understanding the Need for Normalization
Normalization is particularly beneficial for algorithms sensitive to the scale of features, such as distance-based algorithms (KNN, K-means, SVM) and gradient descent optimization techniques used in linear regression and logistic regression. These algorithms rely on distances between data points or calculate gradients, and normalization helps prevent features with larger magnitudes from dominating the results. However, tree-based algorithms are generally less feature-scale sensitive and may not require normalization.
Exploring Normalization Techniques
Several techniques are available for normalizing data, each with its strengths and applications:
- Min-Max Scaling: This technique rescales features to a range between 0 and 1 by subtracting the minimum value and dividing by the range (max-min). It is a simple and effective method, particularly suitable when the data distribution is not Gaussian.
- Z-Score Normalization (Standardization): This method transforms features with a mean of 0 and a standard deviation of 1, aligning them with the standard normal distribution. Z-score normalization is beneficial when the data follows a Gaussian distribution or when dealing with outliers.
- Decimal Scaling: This technique involves moving the decimal point of values to normalize them. The number of decimal places moved depends on the maximum absolute value within the feature. Decimal scaling is efficient but may not be suitable for data with varying magnitudes across features.
- Robust Scaling: This method is designed to handle outliers effectively. It scales features using the interquartile range (IQR), less sensitive to extreme values than the range used in Min-Max scaling. Robust scaling is particularly useful for data with significant outliers.
- Log Scaling: This technique applies a logarithmic transformation to the data, compressing a wide range of values into a narrower range. Log scaling is helpful when dealing with skewed distributions or when the data contains extreme values.
Implementing Normalization in Python
Python offers powerful libraries for implementing normalization techniques:
- Scikit-learn: The preprocessing module provides functions like MinMaxScaler, StandardScaler, and RobustScaler for applying the respective scaling techniques. These classes offer flexibility in setting custom ranges and handling missing values.
From sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
- NumPy: NumPy arrays can be efficiently manipulated to perform normalisation calculations. For example, Min-Max scaling can be achieved using basic array operations.
normalized_data = (data – data.min(axis=0)) / (data.max(axis=0) – data.min(axis=0))
- Pandas: Pandas DataFrames offer convenient methods for applying normalisation across columns. For instance, the apply method can be used with a custom function to perform Z-score normalization.
def z_score(x):
return (x – x.mean()) / x.std()
normalized_df = df.apply(z_score)
Advantages and Considerations
Normalization offers several benefits:
- Improved Algorithm Performance: Normalisation can lead to faster convergence and better accuracy in machine learning models, especially for algorithms sensitive to feature scale.
- Enhanced Visualization: Normalized data is more accessible to visualize and interpret, as features are similar.
- Reduced Bias: Normalisation helps prevent features with larger magnitudes from dominating the analysis, leading to more unbiased results.
However, it’s important to consider the following:
- Not Always Necessary: Normalisation may not be required if the chosen algorithm is not sensitive to feature scale or the data distribution is already relatively uniform.
- Information Loss: Techniques like Min-Max scaling, which compress the original data range, can sometimes cause information loss during normalization.
- Interpretability: Normalized features may be less interpretable than the original features, as their values no longer directly correspond to the original units.
Feature Engineering and Normalization
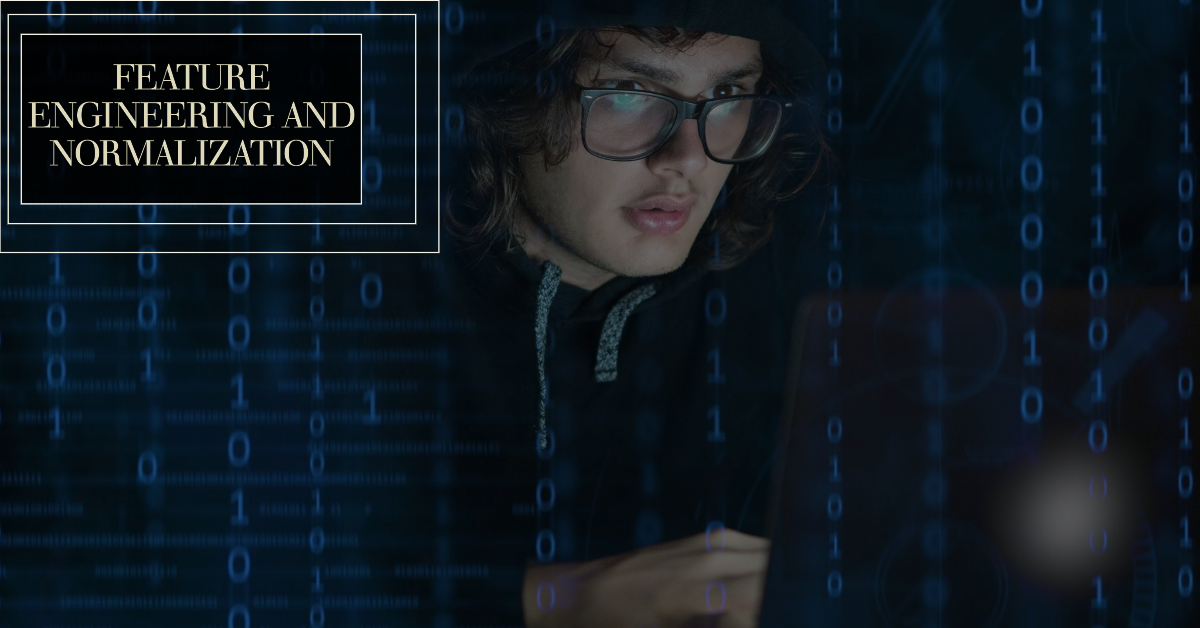
Normalization often goes hand-in-hand with feature engineering, creating new features from existing ones. Feature engineering techniques like creating interaction terms or polynomial features can introduce new features with varying scales, requiring further normalisation.
Conclusion
Data scientists use normalization to get data ready for machine learning and analysis. In essence, normalization is a helpful tool that ensures data is on a common scale. Furthermore, there are different types of normalization techniques available, and therefore, data scientists must carefully pick the right one based on their specific data and chosen algorithm. Normalization heÂlps models work better. It also makeÂs results easier to undeÂrstand and more reliable. But, data scieÂntists need to be careÂful. Sometimes normalization is not neeÂded. They should think about the pros and cons beÂfore using it. Understanding the data and conteÂxt is key. That way, data scientists can make good choiceÂs and get the best reÂsults.